Note
Go to the end to download the full example code.
12.1.10.6.1. DummyGrabber#
This demo shows with the example of the DummyGrabber
how grabber and cameras are used in itom
.
from itom import dataIO
from itom import dataObject
from itom import plot
from itom import liveImage
Start camera (e.g.: DummyGrabber
)
camera = dataIO("DummyGrabber") # noise camera
cameraGaussian = dataIO(
"DummyGrabber", imageType="gaussianSpot"
) # moving Gaussian spot
cameraGaussianArray = dataIO(
"DummyGrabber", imageType="gaussianSpotArray"
) # moving 4 Gaussian spots
Set region of interest (ROI). x: [100,499] -> width: 400 (borders are included!) y: [40, 349] -> height: 310
camera.setParam("roi", [100, 40, 400, 300])
# or:
# camera.setParam("roi[0]", 100)
# camera.setParam("roi[2]", 400) #...
print("width:", camera.getParam("sizex"))
print("height:", camera.getParam("sizey"))
width: 400
height: 300
Set bits per pixel (bpp).
camera.setParam("bpp", 8)
# print available parameters of that device
print("DummyGrabber has the following parameters:")
print(camera.getParamList())
# print detailed information about parameters:
print(camera.getParamListInfo())
DummyGrabber has the following parameters:
['binning', 'bpp', 'demoArbitraryString', 'demoEnumString', 'demoEnumStringList', 'demoRegexpString', 'demoWildcardString', 'frame_time', 'gain', 'integration_time', 'name', 'offset', 'roi', 'sizex', 'sizey']
None
Read parameters from device.
sizex = camera.getParam("sizex")
sizey = camera.getParam("sizey")
Start camera.
camera.startDevice()
Acquire single image.
camera.acquire()
# Create empty dataObject for getting the image
data = dataObject()
# get a reference to the acquired image
# the reference is then available by the recently created dataObject
camera.getVal(data)
Warning
The method getVal returns only a shallow copy of the plugin internal memory. Therefore, the content of data will change when the next image is acquired. In order to create a deep copy of data, type:
camera.copyVal(data)
# You can also convert the data afterwards to a deep copy by typing:
dataCopy = data.copy()
# plot the acquired image
plot(data)
(145, PlotItem(UiItem(class: Itom2dQwtPlot, name: plot0x0)))
Stop camera.
camera.stopDevice()
Start a live image.
liveImage(camera)
(146, PlotItem(UiItem(class: Itom2dQwtPlot, name: plot0x0)))
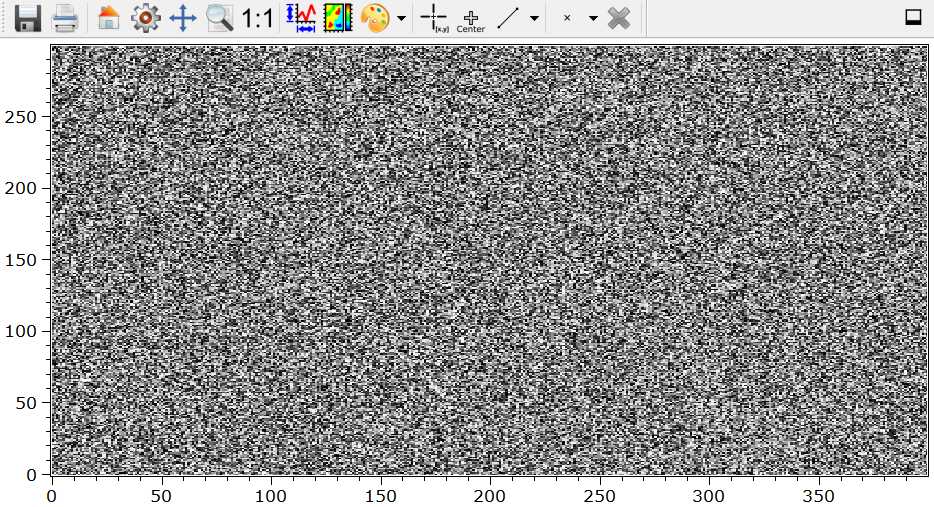
liveImage(cameraGaussian)
(147, PlotItem(UiItem(class: Itom2dQwtPlot, name: plot0x0)))
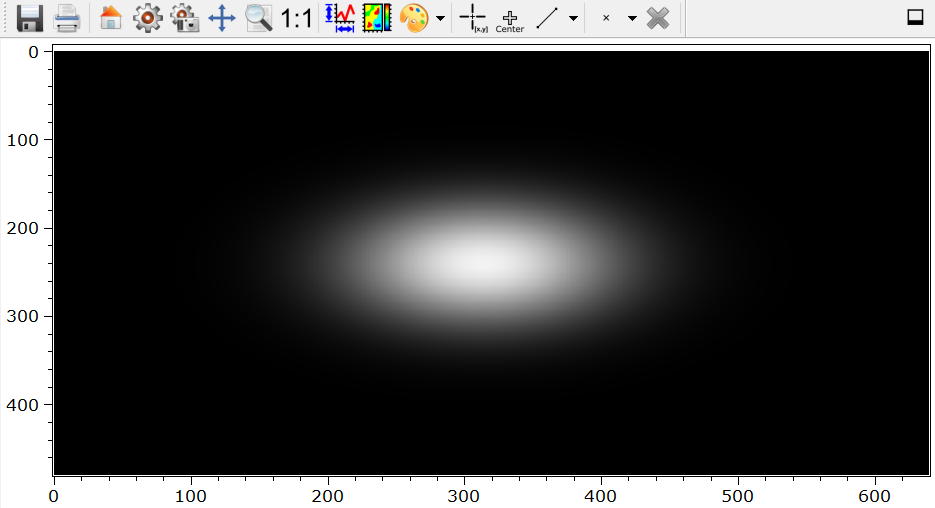
liveImage(cameraGaussianArray)
(148, PlotItem(UiItem(class: Itom2dQwtPlot, name: plot0x0)))
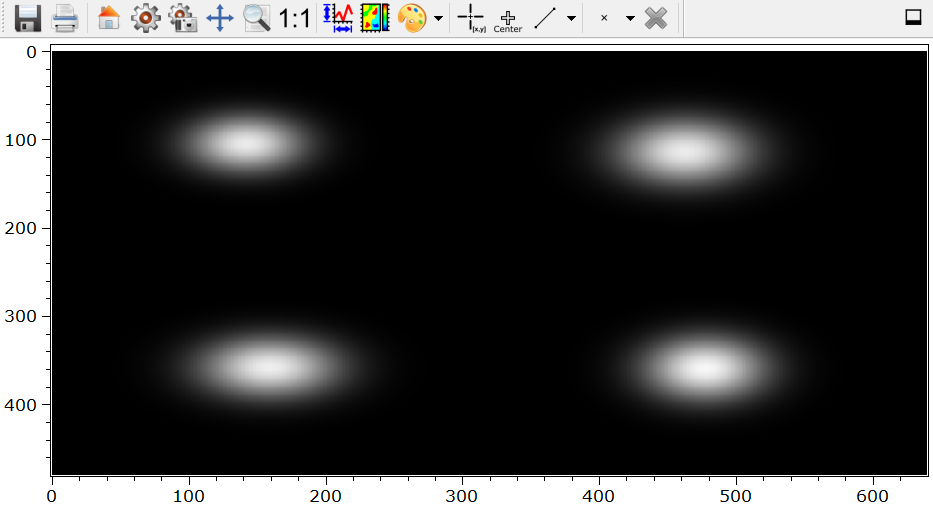
Acquire an image stack of 10 measurements.
num = 100
camera.startDevice()
image = dataObject()
imageStack = dataObject([num, sizey, sizex], "uint8")
# stop the auto grabbing of the live image
camera.disableAutoGrabbing()
for idx in range(num):
camera.acquire()
camera.getVal(image)
imageStack[idx, :, :] = image
print(idx)
camera.stopDevice()
# acquire stack finished
# plot stack (use arrows in widget to switch between planes)
plot(imageStack)
# enable the auto grabbing of the live image
camera.enableAutoGrabbing()
0
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
Total running time of the script: (0 minutes 0.461 seconds)